It is useful to store the images used by a program inside the executable as resources. Visual studio offers an efficient way to do that.
The method is very simple but I always forget how to do it. So, this is a very short tutorial.
Note: I use Visual studio 2017 but the procedure is similar with other versions.
1. Create the project
2. Put the resource in the project
Open the Resource.resx file by double clicking on it. (Visual studio will open a specific editor)
In the toolbar of this editor select Add Resource and select “Add existing file” then choose the desired image file (here I will use summer.bmp).
3. Use the image
You can now use the image resource in the designer i.e. as the image property of a Picture box
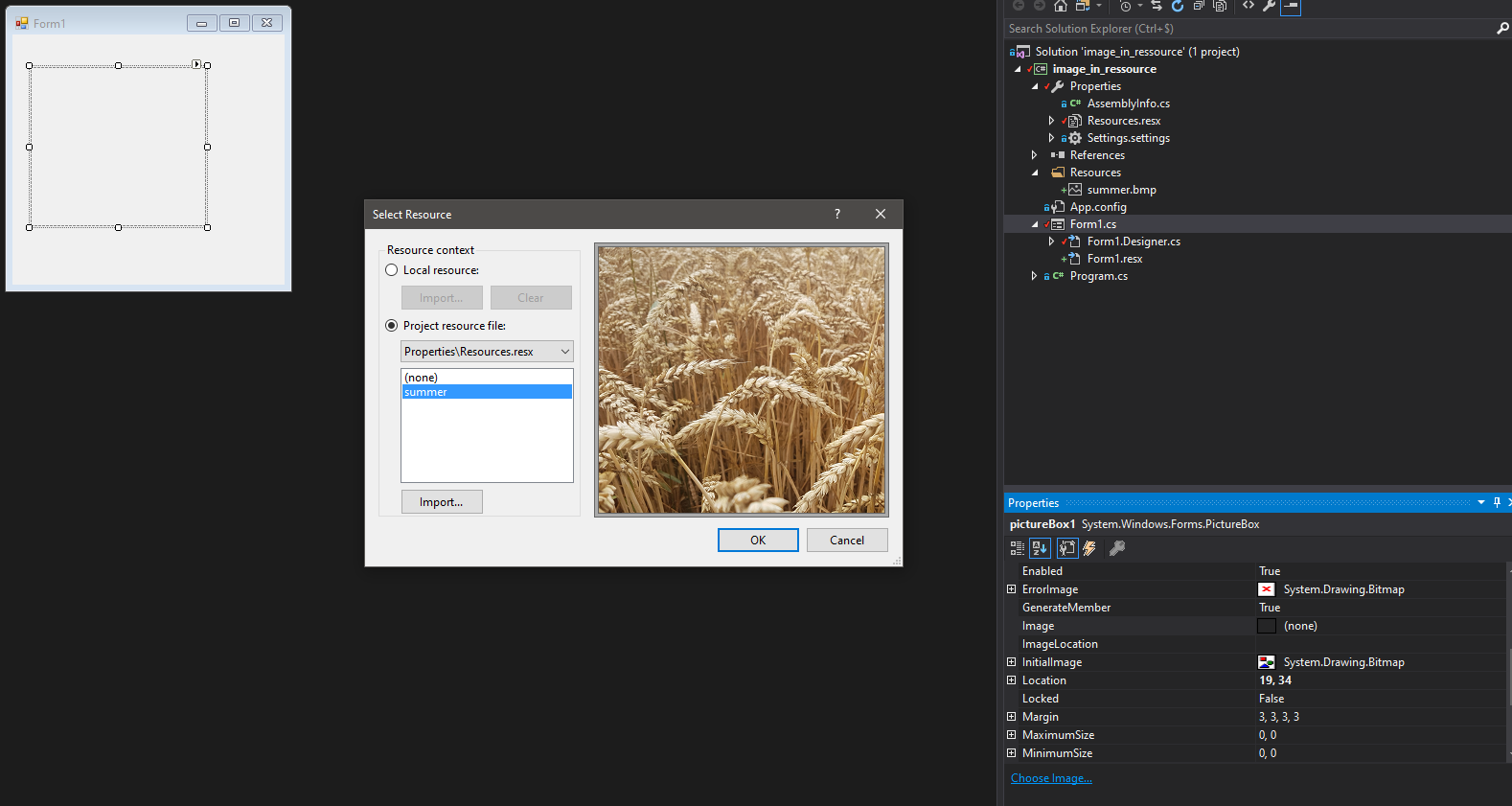
But you can also access the image in the code by using <program_name>.Properties.Resources.<resourcename>.
See example below:
namespace image_in_ressource
{
public partial class Form1 : Form
{
private Image summerImage;
public Form1()
{
InitializeComponent();
summerImage = new Bitmap(image_in_ressource.Properties.Resources.summer);
}
private void Form1_Paint(object sender, PaintEventArgs e)
{
e.Graphics.DrawImageUnscaled(summerImage, new Point(0, 0));
}
}
}